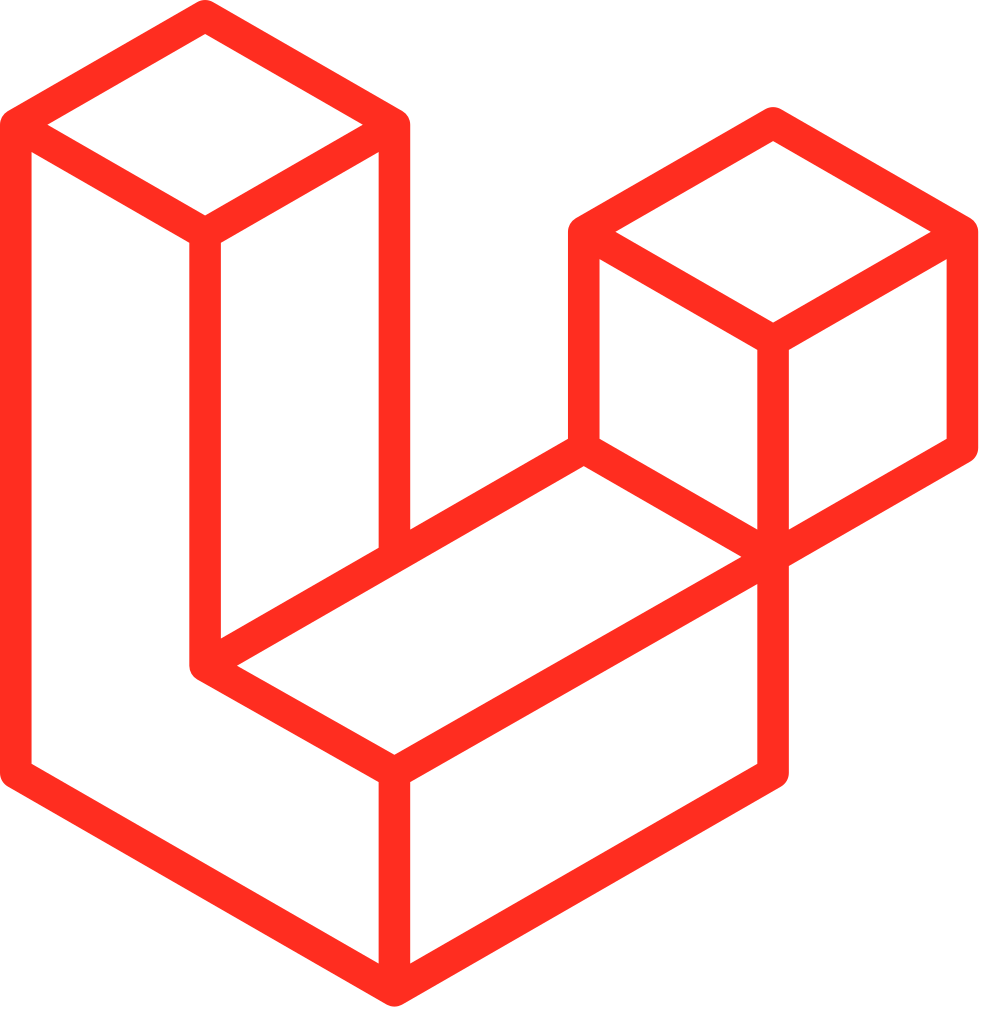
LARAVEL SECURITY BEST PRACTICES
Laravel is a popular PHP development platform that is well known for its clean design and the active user community. Laravel is fairly secure by default because whenever a loophole is discovered, the maintenance team addresses it quickly. But, like any other software platform it’s only as secure as the implementation.
Laravel being a development framework, will not secure your servers or operating system. It will only help secure the application being built. The focus of this article is to provide the security best practices for the implementation of a Laravel framework. In this article, we will cover some of the best practices when it comes to securing your Laravel application.
Laravel Authentication System
Many web applications provide a way for their users to authenticate with the application and "login". Laravel provides tools to implement authentication quickly, securely, and easily. Through the use of ‘guards’ and ‘providers’ as its tools, login security is built right in. Guards define how users are authenticated for each request. For example, Laravel ships with a session guard which maintains state using session storage and cookies. Providers assists in bringing the users session back from the data storage. Using these tools will help ensure secure logins.
To further easy the implementation of a login framework, there are also Laravel Starter Kits with much of the login framework built and ready to go.
Protection against XSS, SQL Injection, and CSRF
Cross Site Scripting (XSS)
During XSS attacks, the attacker enters JavaScript into your website typically through a text form for a blog or some other input. This script on the page, whenever new visitors will access the affected page, the script will be executed with malicious impact.
Laravel's Blade templating engine offers native support of echo statements {{ }} that automatically escape variables using the htmlspecialchars PHP function to protect against XSS attacks so any commands are outputted as HTML instead of executing on the page.
SQL Injection
Laravel’s Eloquent ORM (object relational mapping) uses PDO (PHP Data Objects) binding that protects from SQL injections. Similar to the XSS example above, this feature will alter the strings that get inserted into the database ensuring the intent of your SQL queries can not be modified externally.
As an example, a search form pull records based upon users’ email address from a database. While normally the form would get an email address, but if the user typed in something like “sample@example.com' or 1=1”, the SQL query is modified to:
SELECT * FROM users WHERE email ='sample@example.com' or 1=1
This would allow for all the users to be retrieved by SQL injection.
When the PDO parameter binding is in place, the input is in quotes and the query will look like:
SELECT * FROM users WHERE email = 'sample@example.com or 1=1'
Since no records will match with either the email or the “1=1”, the query will not return anything.
Laravel provides other ways of talking to databases, such as raw SQL queries. But both Eloquent ORM and the Query Builder provide automatic protection against SQL injections by adding param binding by default.
Cross-Site-Request-Forgery (CSRF)
For the purpose of protecting the system from third parties trying to generate faulty requests externally, Laravel Security utilizes CSRF tokens.
Whenever a request comes from a submitted form or through an AJAX call, this platform creates and then combines an appropriate token into it. In Blade, you can use the directive @csrf to generate this token.
When this occurs, the Laravel Security Scanner tries to figure out if the saved request during a user’s session is the same second time around.
In case the token is not a match, the security features invalidate the request automatically and cancel the command.
Protecting Cookies, Password Vulnerabilities, Laravel Encryption and Hashing
Like many secured sources of data one would want to keep passwords and potentially data in cookies private. Thus there is data in these systems that can be stored in a way to make it unreadable if obtained by an unwanted hacker. The two systems which are part of the Laravel framework for this are hashing and encryption.
Both hashing and encryption take the plain text data and convert it into a form that is not easily transformed back into its original form. The default hash mechanism in Laravel, uses Argon2 and Bcrypt. This hashing function protects the sensitive data and all passwords properly. The Laravel encryption services provide an additional level of security over hashing by the use of a message authentication code (MAC). The MAC provides an additional check so that that their underlying value can not be modified or tampered with once encrypted.
With these mechanisms passwords and cookies can store private information that is not easily obtained even if captured by another.
Prevent DOS (Denial of Service) Attack
DOS attacks are continue to become more prevalent. These are attached where hackers will send a high volume of requests to disrupt service or use brute force attacks to get into the server with many combinations. These types of attacks can be divided into two popular categories:
DOS Attacks That Send a Lot of Requests
These attacks would send a lot of web requests that try to keep the connection open for as long as possible. The server memory eventually gets full, resulting in our server going down. One example of this is a slow loris attack.
Laravel has a built in Rate Limiter to help us handle these attacks by IP. This helps lessen the impact of these types of attacks. However, it’s often used in conjunction with tools like Fail2Ban to stop requests earlier at the server's firewall level to lessen the load at the application level.
Another example is when a lot of requests are sent to a form trying many combinations to hack into or disrupt the server. To help avoid malicious requests from bots, you can set a hidden input. The bots would fill the input (a normal user should not fill a hidden input), and then you can use the prohibited validation rule from Laravel validator:
// this input should never comes in the request 'honey_pot_field' => ['prohibited'],
DOS Attacks That Send Large Files to Consume the Server Memory
Another variety of a DOS attack could be in a public form to submit a file. Having many large files submitted can exhaust the server memory.
To handle this attack, you can use the Laravel API security validator to validate the file from the request. Here is an example:
// file max size is 512 kilobytes.. 'photo' => ['mimes:jpg,bmp,png', 'file', 'max:512']
Conclusion
While Laravel has some security features built in, they need to be used and implemented to make the system secure. This introduction to the security aspects of Laravel allows you to get a better understanding of what’s available. We hope this provides a useful guide to making your Laravel systems a little bit more secure.